AMPscript Format Functions
AMPscript DateTime functions allow us to manipulate display format, perform math functions, and control email content using static, dynamic, and real-time values. Formatting functions provide a powerful tool set for converting various data types to formatted strings and numbers.
- FormatDate(1, 2, 3, 4)
- FormatCurrency(1, 2, 3, 4)
- FormatNumber(1, 2, 3)
- Format(1, 2, 3, 4)
Format Date Function
This function formats a specified string as a date value for a specified format pattern and locale.
Example
%%=FormatDate(1, 2, 3, 4)=%%
Format Currency Function
This function formats a specified string as a currency value for a specified locale.
Example
%%=FormatCurrency(1, 2, 3, 4)=%%
Format Number Function
This function formats a specified string as a number for a specified locale.
Example
%%=FormatNumber(1, 2, 3)=%%
Format Function
This function formats a string into a specified format pattern and locale. You’ll want to use this function for date and time formatting that requires a locale setting.
Example
%%=Format(1, 2, 3, 4)=%%
Gallery
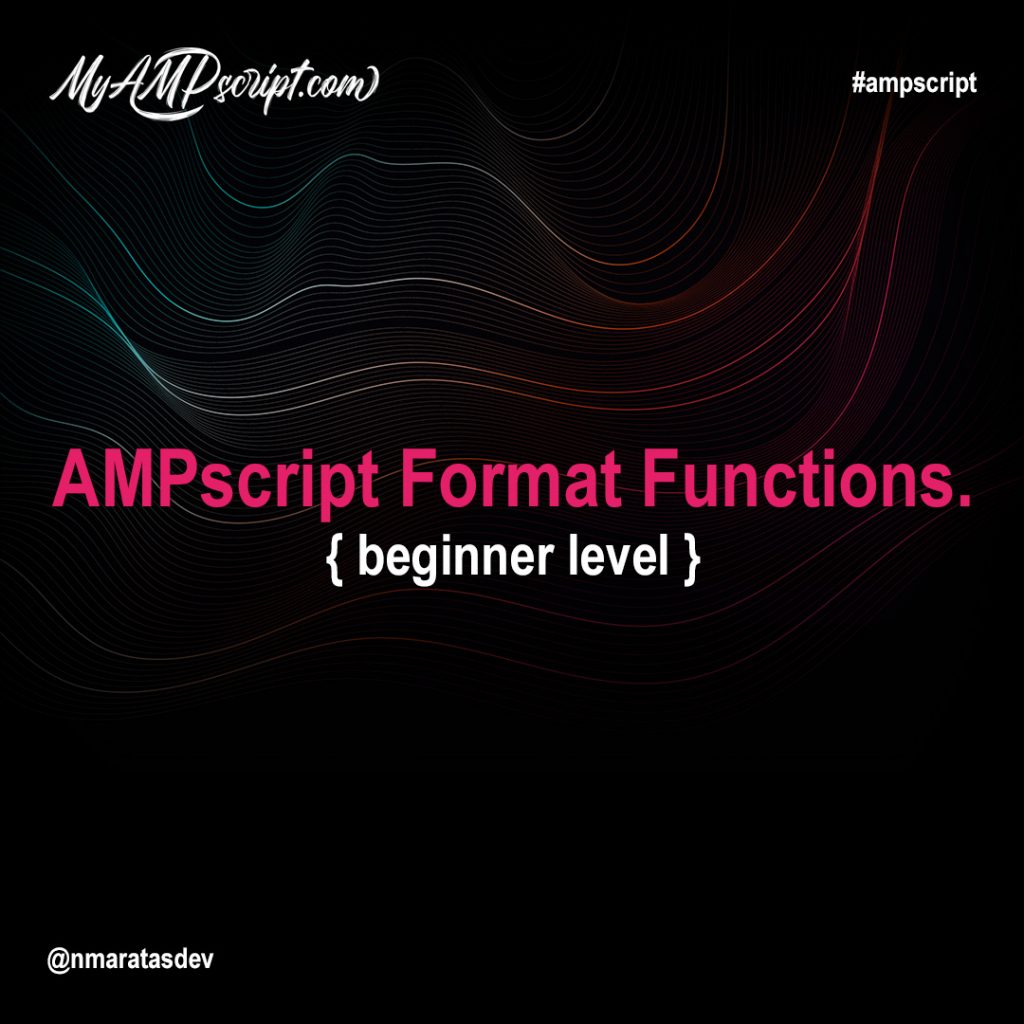
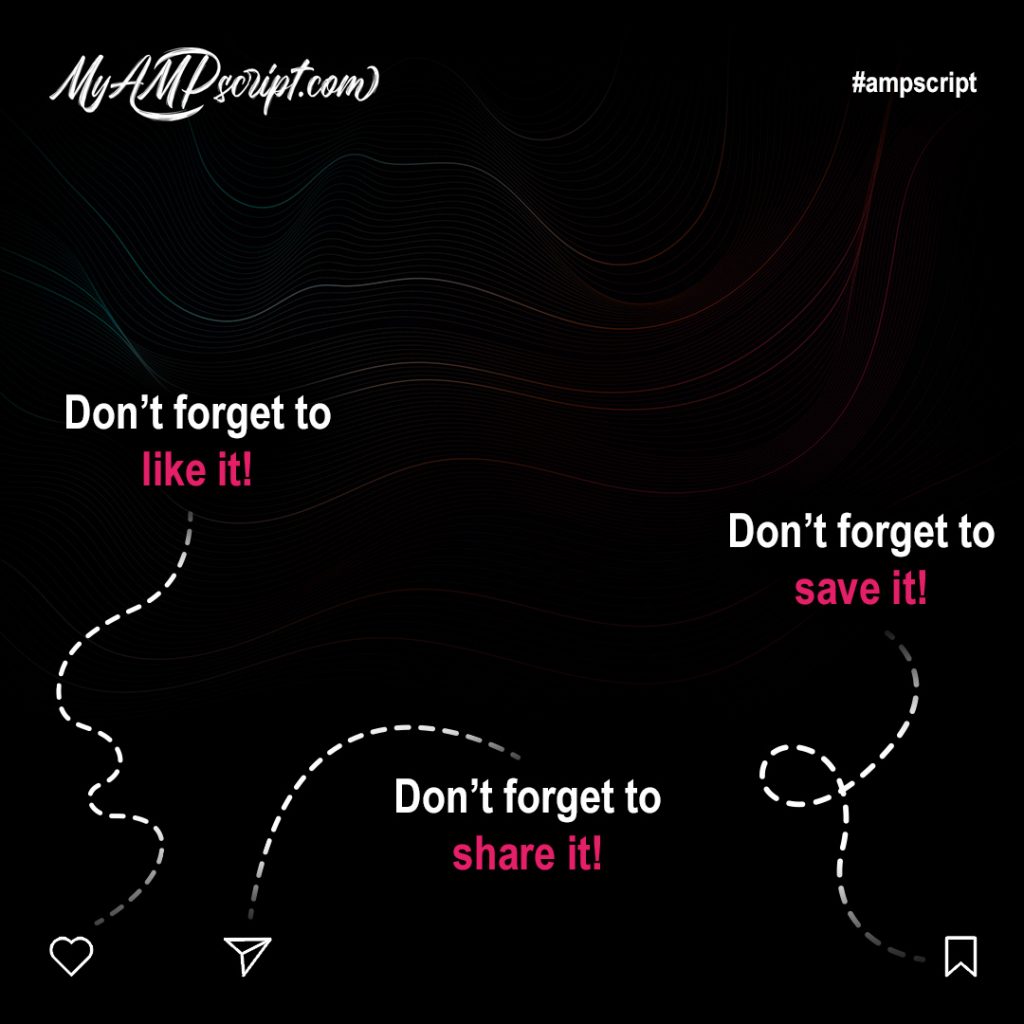
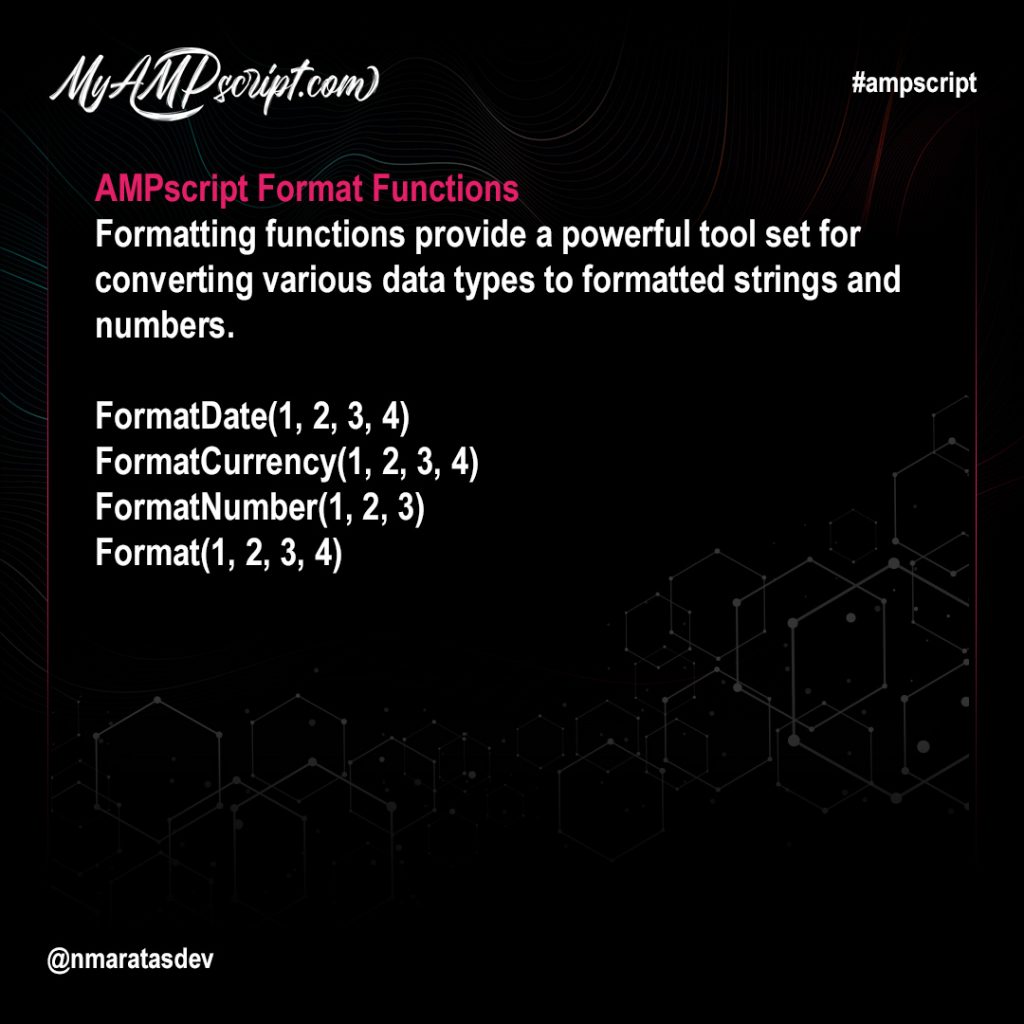
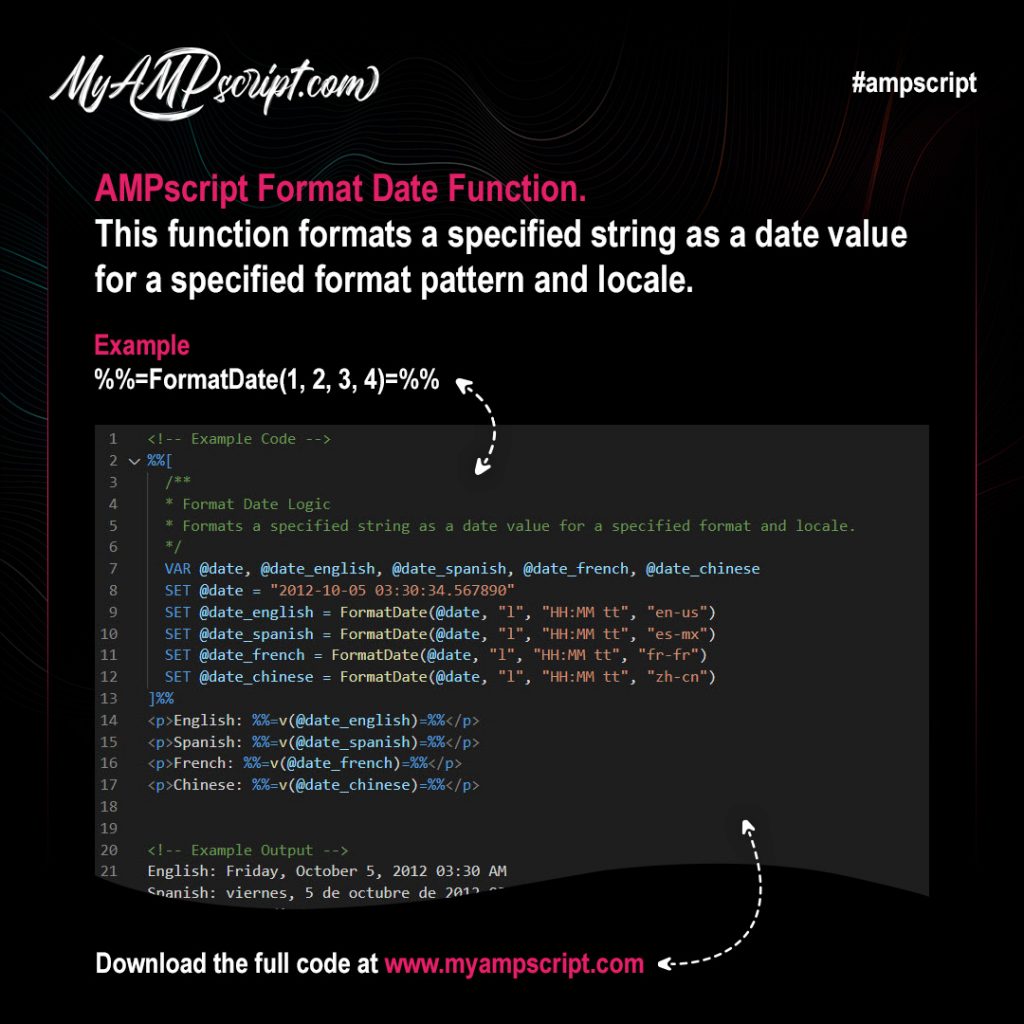
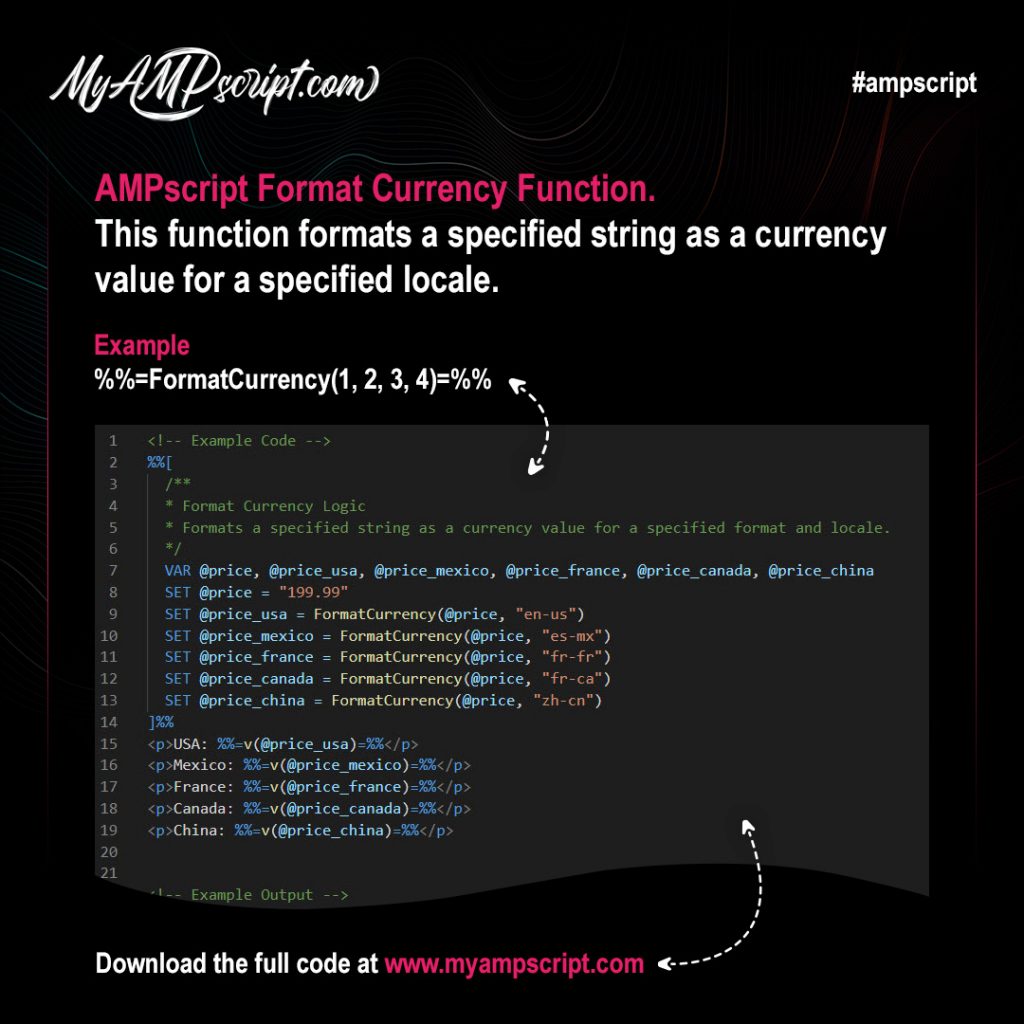
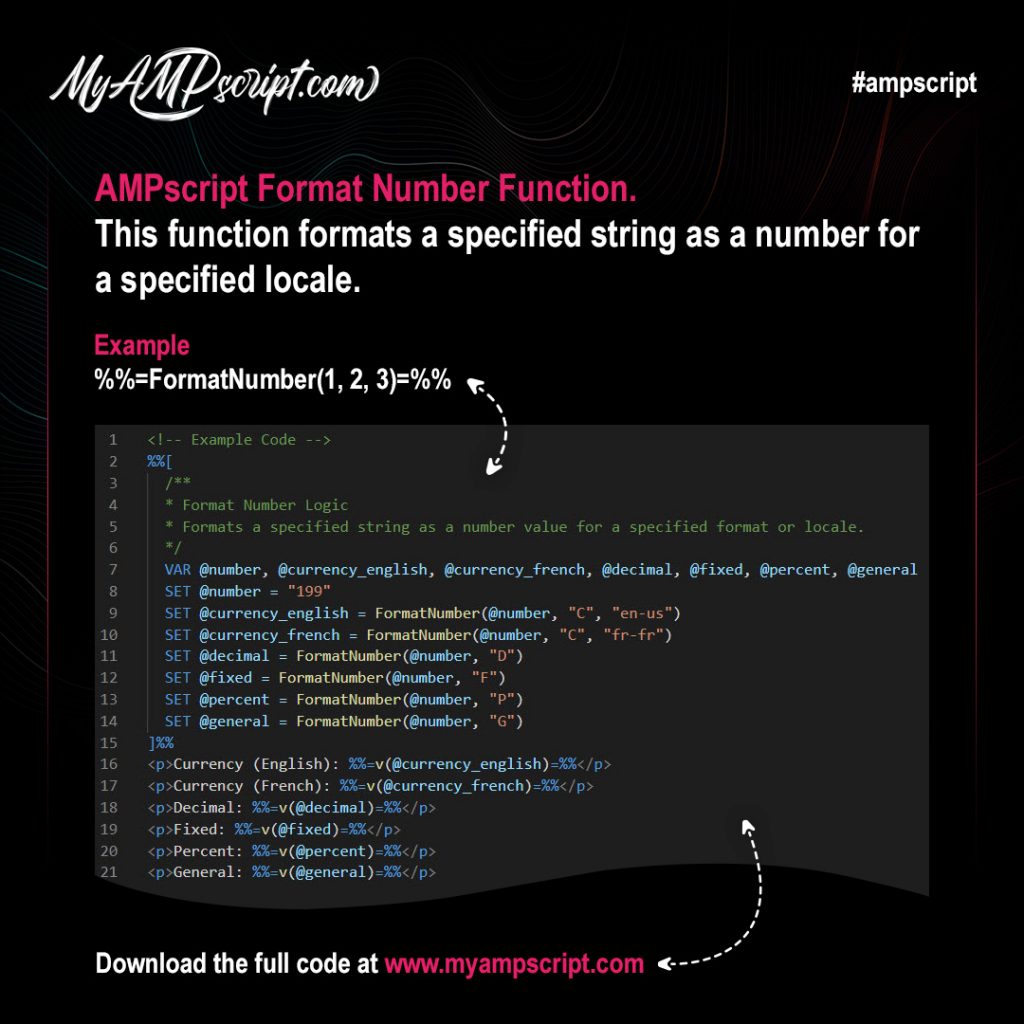
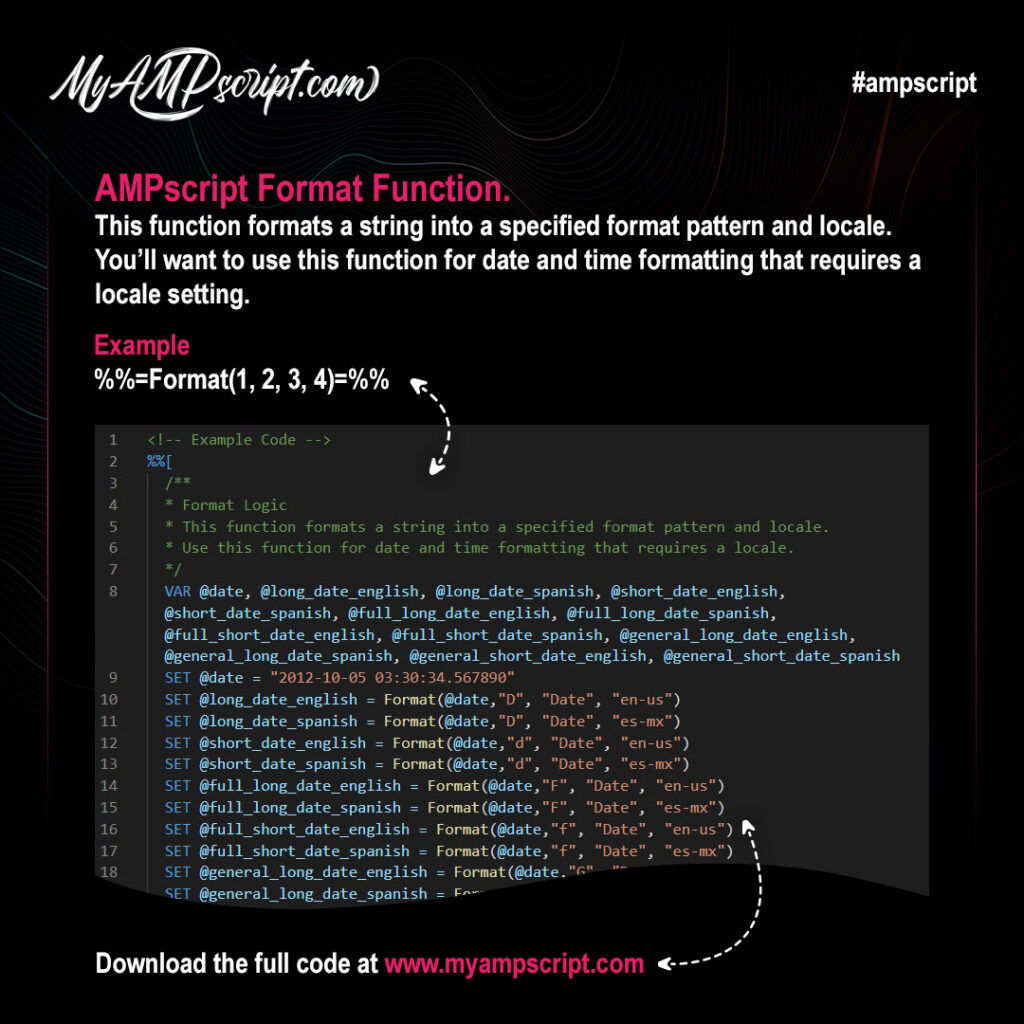
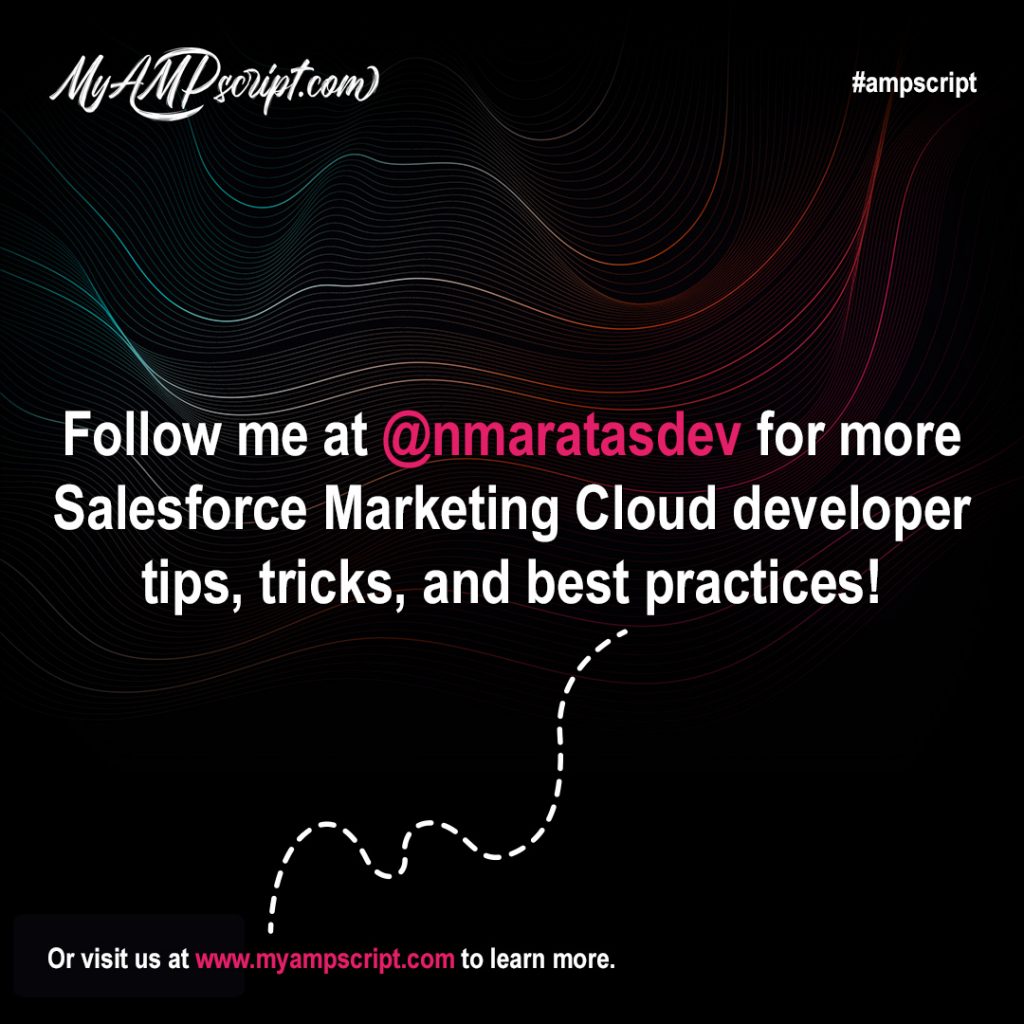
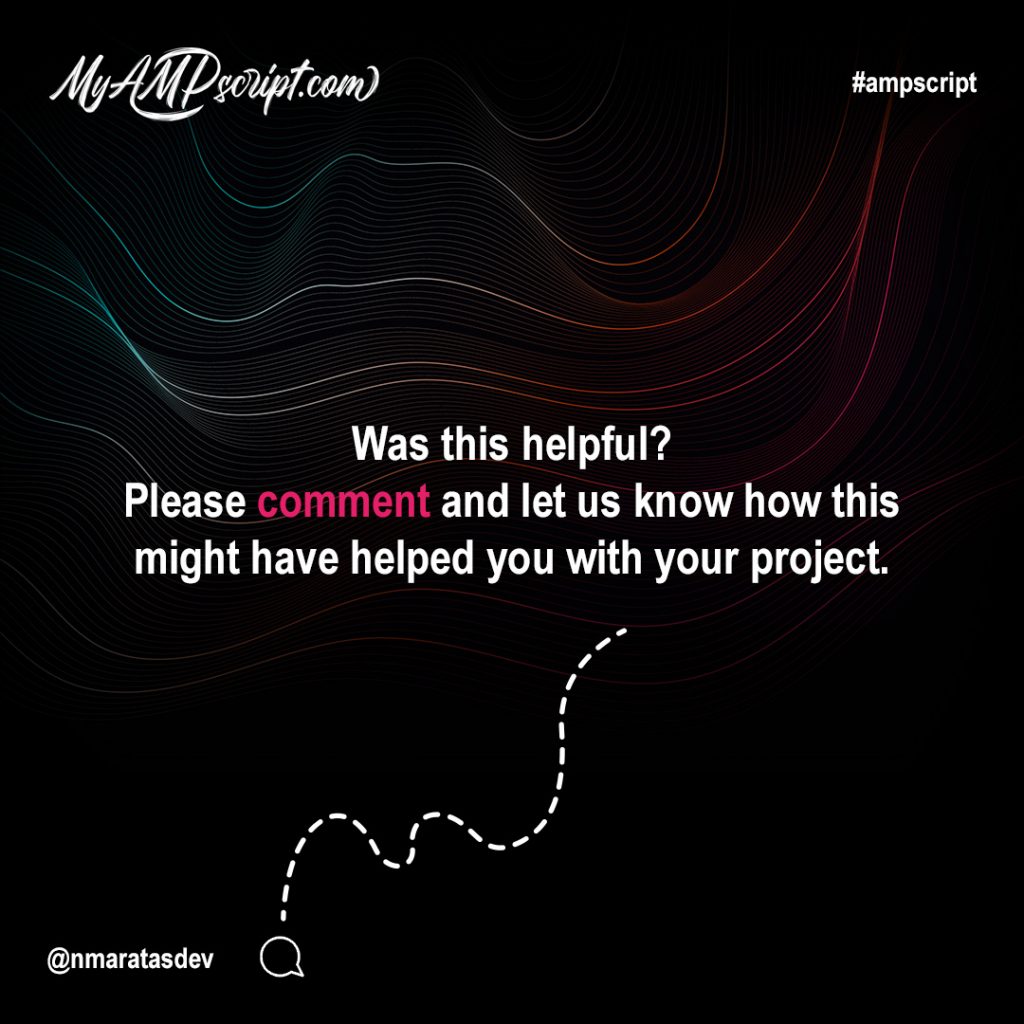
Hi Everyone! I hope this information helps. You can download the full code using the links below. Feel free to modify it to fit your needs. I’d love to get your feedback, did this information help in some way?
AMPscript Format Date Code
https://gist.github.com/nmaratasdev/3ebe1a423071ad93fd4fbddb9b7737a7
AMPscript Format Currency Code
https://gist.github.com/nmaratasdev/bf3a8d605b4bed2b073c71f9809482d6
AMPscript Format Number Code
https://gist.github.com/nmaratasdev/f13420c8eb25f15c7711fe2ae220a791
AMPscript Format Code
https://gist.github.com/nmaratasdev/f5e4ea089d39f2a729f69f4c64ace9c4